This page was generated from notebooks/05_Greedy_coloring.ipynb.
Interactive online version:
Getting started with mapclassify.greedy¶
Greedy or topological coloring (or sequential coloring) is a cartographic method of assigning colors to polygons (or other geometries, mapclassify.greedy
supports all geometry types) in such a way, that no two adjacent polygons share the same color.
greedy
is a small toolkit within mapclassify
providing such a functionality on top of GeoPandas GeoDataFrames. mapclassify.greedy()
is all we need.
[4]:
from mapclassify import __version__, greedy
__version__
[4]:
'2.6.0+5.g2788475.dirty'
[5]:
import geopandas
import seaborn
seaborn.set()
Few of greedy
’s methods of coloring require either measuring of areas or distances. To obtain proper values, our GeoDataFrame needs to be in a projected CRS. Let’s use Africa and reproject it to ‘ESRI:102022’:
[6]:
world = geopandas.read_file("https://naciscdn.org/naturalearth/110m/cultural/ne_110m_admin_0_countries.zip")
africa = world.loc[world.CONTINENT == "Africa"]
africa = africa.to_crs("ESRI:102022")
ax = africa.plot(figsize=(8, 12), edgecolor="w")
ax.set_axis_off()
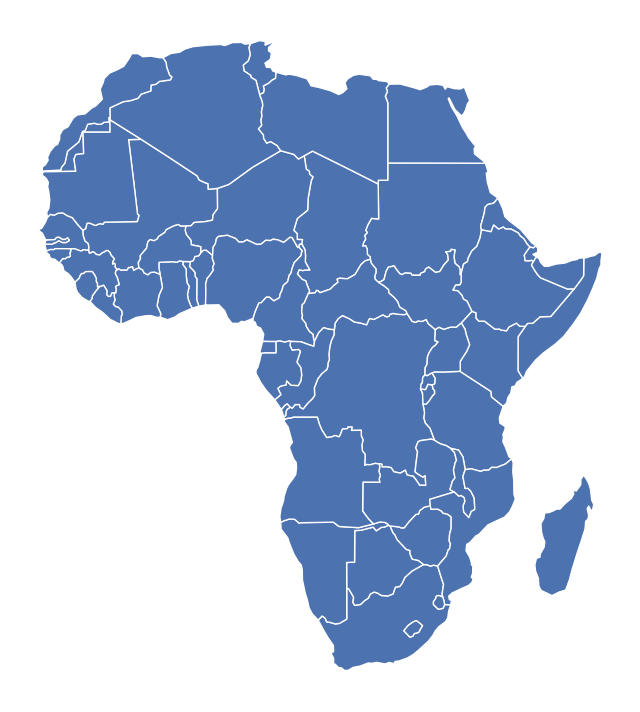
Default¶
The default usage of greedy
is extremely simple. Greedy returns a Series with color codes, so we can assign it directly as a new column of our GeoDataFrame:
[7]:
africa["greedy_default"] = greedy(africa)
africa["greedy_default"].head(5)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
[7]:
1 1
2 0
11 0
12 1
13 4
Name: greedy_default, dtype: int64
Using resulting color codes as plotting categories gives us following the plot:
[8]:
ax = africa.plot(
figsize=(8, 12),
column="greedy_default",
categorical=True,
cmap="Set3",
legend=True,
edgecolor="w",
)
ax.set_axis_off()
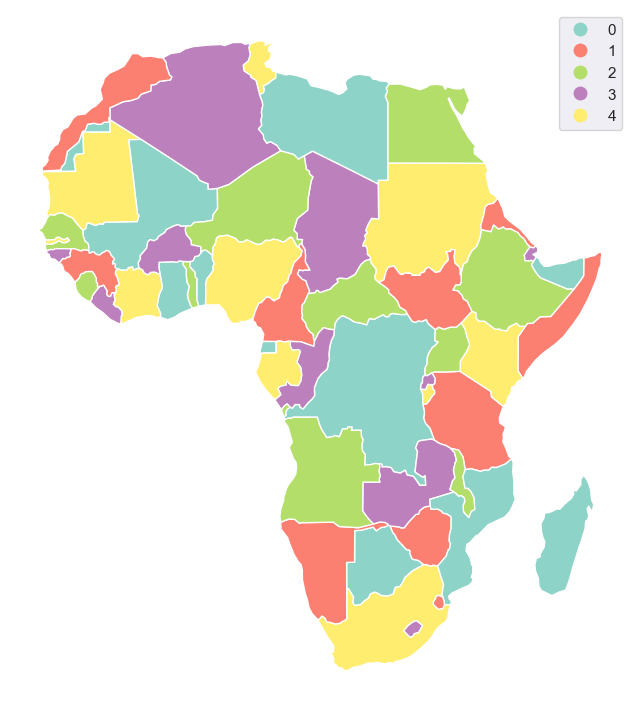
Strategies¶
Balanced¶
Greedy offers several strategies of coloring. The default strategy is balanced
based on count
attempting to balance the number of features per each color. Other balanced modes are area
(balance the area covered by each color), distance
and centroid
(both attemtps to balance the distance between colors). Each of them attempt to balance the color assignment according to different conditions and hence can result in a different number of colors.
[9]:
africa = africa.reset_index(drop=True)
[10]:
africa["greedy_area"] = greedy(africa, strategy="balanced", balance="area")
ax = africa.plot(
"greedy_area",
categorical=True,
figsize=(8, 12),
cmap="Set3",
legend=True,
edgecolor="w",
)
ax.set_axis_off()
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
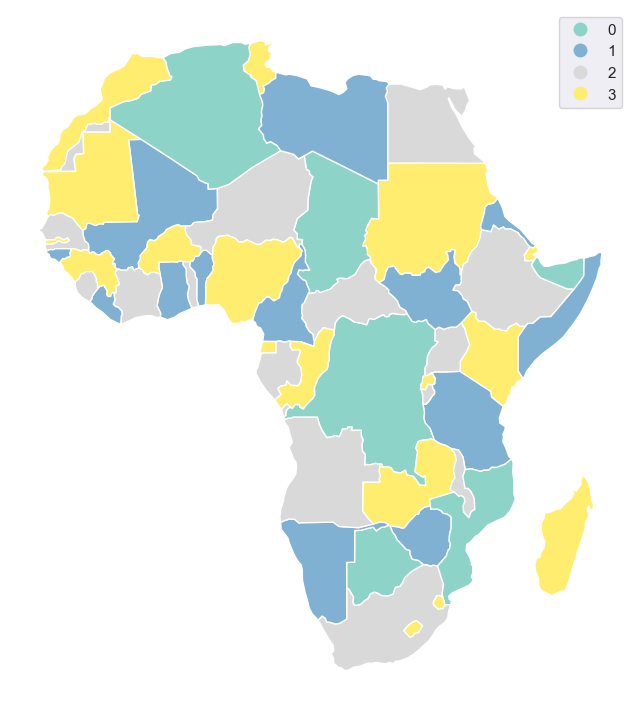
Different modes of balancing within the balanced
strategy can be set using the balance
keyword.
[11]:
africa["greedy_distance"] = greedy(africa, strategy="balanced", balance="distance")
ax = africa.plot(
"greedy_distance",
categorical=True,
figsize=(8, 12),
cmap="Set3",
legend=True,
edgecolor="w",
)
ax.set_axis_off()
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
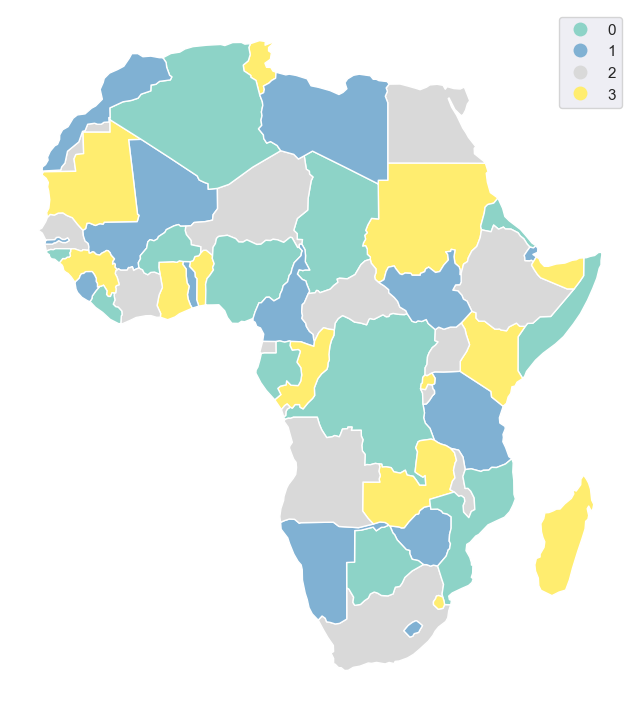
NetworkX strategies¶
On top of four modes of balanced coloring strategy, greedy
offers all networkx.greedy_coloring()
strategies, like largest_first
:
[12]:
africa["greedy_largest_first"] = greedy(africa, strategy="largest_first")
ax = africa.plot(
"greedy_largest_first",
categorical=True,
figsize=(8, 12),
cmap="Set3",
legend=True,
edgecolor="w",
)
ax.set_axis_off()
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
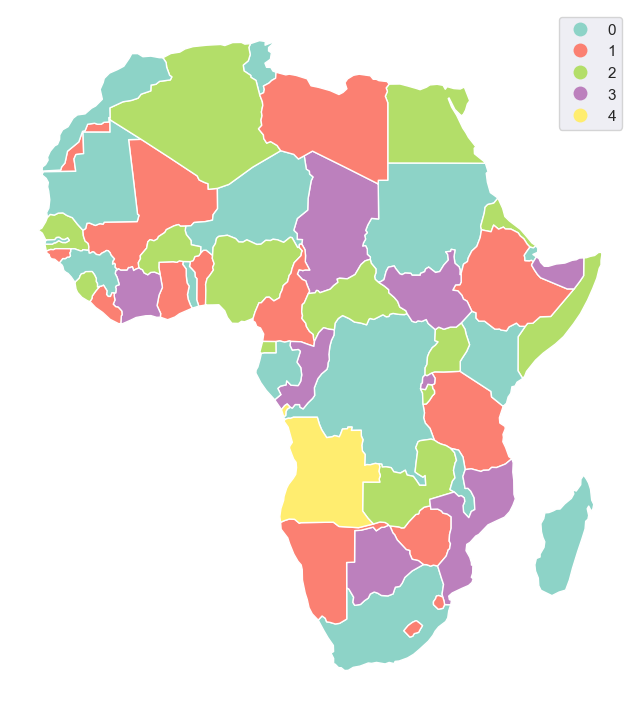
Another strategy provided by NetworkX is smallest_last
. All strategies provide different results. Check Comparison of strategies below for details.
[13]:
africa["greedy_s"] = greedy(africa, strategy="smallest_last")
ax = africa.plot(
"greedy_s",
categorical=True,
figsize=(8, 12),
cmap="Set3",
legend=True,
edgecolor="w",
)
ax.set_axis_off()
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
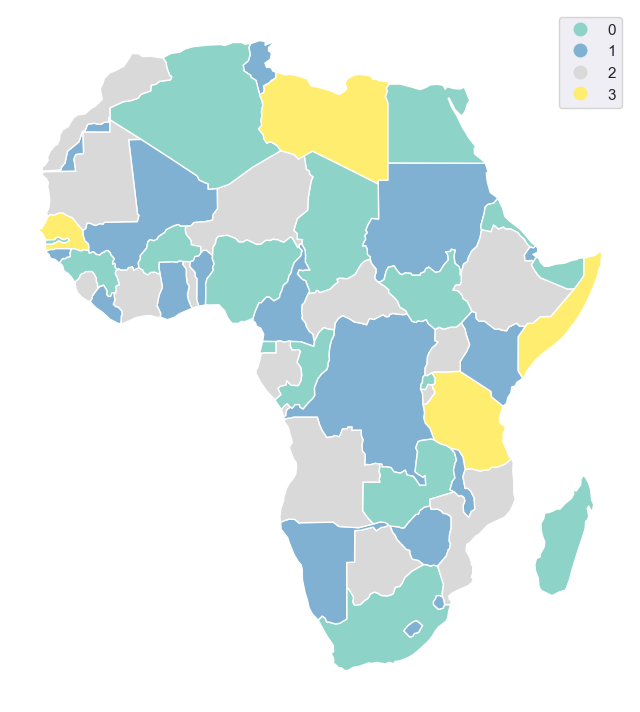
Greedy is variable in a way how to define adjacency and which coloring strategy to use. All options are described in this documentation together with comparison of their performance.
Defining adjacency¶
The key in toplogical coloring is the definition of adjacency, to understand which features are neighboring and could not share the same color. mapclassify.greedy
comes with several methods of defining it. Binary spatial weights denoting adjacency are then stored as libpysal
weights objects.
[14]:
from shapely.geometry import Point
For illustration purposes, let’s generate a 10x10 mesh of square polygons:
[15]:
def poly_lattice_gdf(dim, plot=False):
polys = []
for x in range(dim):
for y in range(dim):
polys.append(Point(x, y).buffer(0.5, cap_style=3))
_gdf = geopandas.GeoDataFrame(geometry=polys)
if plot:
ax = _gdf.plot(edgecolor="w")
ax.set_axis_off()
return _gdf
[16]:
gdf = poly_lattice_gdf(10, plot=True)
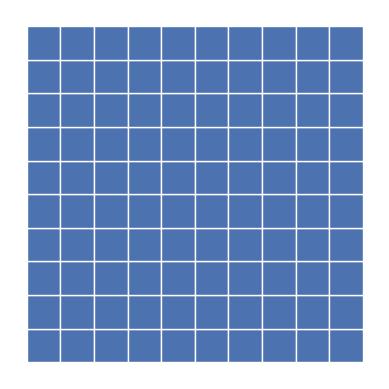
libpysal adjacency¶
The most performant way of generating spatial weights is using libpysal contiguity weights. As they are based on the shared nodes or edges, the dataset needs to be topologically correct. Neighboring polygons needs to share vertices and edges, otherwise their relationship will not be captured. There are two ways to define contiguity weights - "rook"
and "queen"
.
Rook¶
Rook identifies two objects as neighboring only if they share at least on edge - line between two shared points. Use rook if you do not mind two polygons touching by their corners having the same color:
[17]:
gdf["rook"] = greedy(gdf, sw="rook", min_colors=2)
ax = gdf.plot("rook", edgecolor="w", categorical=True, cmap="Set3")
ax.set_axis_off()
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
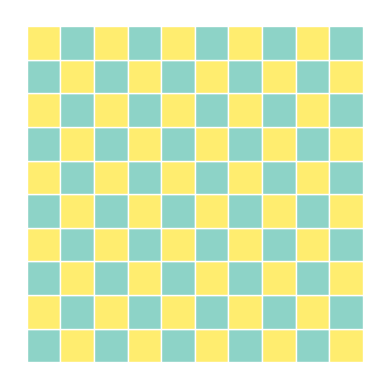
Queen¶
The default option in greedy
is "queen"
adjacency. Queen adjaceny identifies two objects as neighboring if they share at least one point. It ensures that even polygons sharing only one corner will not share a color:
[18]:
gdf["queen"] = greedy(gdf, sw="queen", min_colors=2)
ax = gdf.plot("queen", edgecolor="w", categorical=True, cmap="Set3")
ax.set_axis_off()
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
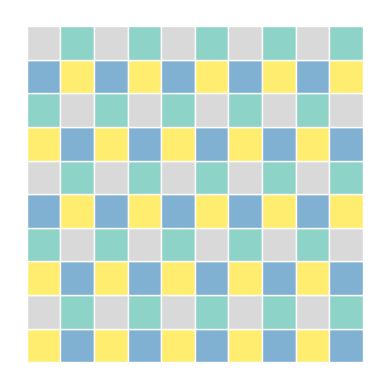
Intersection-based adjacency¶
As noted above, if the topology of the dataset is not ideal, libpysal might not identify two visually neighboring features as neighbors. greedy
can utilize an intersection-based algorithm using GEOS intersection to identify if two features intersects in any way. They do not have to share any points. Naturally, such an approach is significantly slower (details below), but it can provide correct adjacency when libpysal fails.
To make greedy
use this algorithm, one just needs to define min_distance
. If it is set to 0, it behaves similarly to queen
contiguity, just capturing all intersections:
[19]:
gdf["geos"] = greedy(gdf, min_distance=0, min_colors=2)
ax = gdf.plot("geos", edgecolor="w", categorical=True, cmap="Set3")
ax.set_axis_off()
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
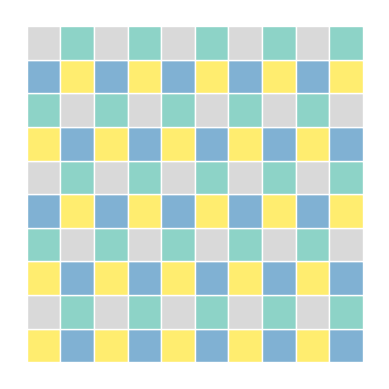
min_distance
also sets the minimal distance between colors. To do that, all features within such a distance are identified as neighbors, hence no two features within the set distance can share the same color:
[20]:
gdf["dist1"] = greedy(gdf, min_distance=1, min_colors=2)
ax = gdf.plot("dist1", edgecolor="w", categorical=True, cmap="Set3")
ax.set_axis_off()
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
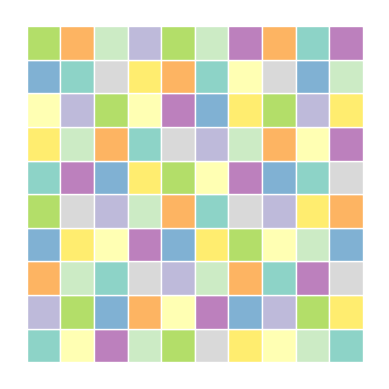
Reusing spatial weights¶
Passing libpysal.weights.W
object to sw
, will skip generating spatial weights and use the passed object instead. That will improve the performance if one intends to repeat the coloring multiple times. In that case, weights should be denoted using the GeodataFrame’s index.
Performance¶
The difference in performance of libpysal and GEOS-based method is large, so it is recommended to use libpysal if possible. Details of comparison between all methods are below:
[21]:
import numpy
import pandas
import time
[22]:
def run_greedy(_gdf, greedy_kws, min_colors=4, runs=5):
timer = []
for run in range(runs):
s = time.time()
colors = greedy(_gdf, min_colors=min_colors, **greedy_kws)
e = time.time() - s
timer.append(e)
_mean_time = round(numpy.mean(timer), 4)
return _mean_time, colors
def printer(m, t, c):
print(f"\t{m}:\t", t, "s;\t", c + 1, "colors")
[23]:
params = {
"rook": dict(sw="rook"),
"queen": dict(sw="queen"),
"geos": dict(min_distance=0),
"dist1": dict(min_distance=1)
}
[24]:
times = pandas.DataFrame(index=params.keys())
steps = range(10, 110, 10)
for step in steps:
print(step, "----------------------------------------------")
gdf = poly_lattice_gdf(step, plot=False)
for method, kwargs in params.items():
mean_time, colors = run_greedy(gdf, kwargs, min_colors=2)
printer(method, mean_time, numpy.max(colors))
times.loc[method, step] = mean_time
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
10 ----------------------------------------------
rook: 0.0031 s; 2 colors
queen: 0.0028 s; 4 colors
geos: 0.0026 s; 4 colors
dist1: 0.0057 s; 10 colors
20 ----------------------------------------------
rook: 0.0107 s; 2 colors
queen: 0.0099 s; 4 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
geos: 0.0061 s; 4 colors
dist1: 0.0188 s; 10 colors
30 ----------------------------------------------
rook: 0.0239 s; 2 colors
queen: 0.0224 s; 4 colors
geos: 0.0131 s; 4 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
dist1: 0.069 s; 10 colors
40 ----------------------------------------------
rook: 0.0462 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.0471 s; 4 colors
geos: 0.0261 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
dist1: 0.0846 s; 10 colors
50 ----------------------------------------------
rook: 0.0698 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.0683 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
geos: 0.048 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
dist1: 0.1344 s; 10 colors
60 ----------------------------------------------
rook: 0.1067 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.1031 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
geos: 0.0729 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
dist1: 0.2205 s; 10 colors
70 ----------------------------------------------
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
rook: 0.1493 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.1396 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
geos: 0.1082 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
dist1: 0.3423 s; 10 colors
80 ----------------------------------------------
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
rook: 0.1976 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.1864 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
geos: 0.1639 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
dist1: 0.4713 s; 10 colors
90 ----------------------------------------------
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
rook: 0.27 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.2479 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
geos: 0.2187 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
dist1: 0.646 s; 10 colors
100 ----------------------------------------------
/Users/martin/Git/mapclassify/mapclassify/greedy.py:308: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Rook.from_dataframe(gdf, silence_warnings=silence_warnings)
rook: 0.3191 s; 2 colors
/Users/martin/Git/mapclassify/mapclassify/greedy.py:306: FutureWarning: `use_index` defaults to False but will default to True in future. Set True/False directly to control this behavior and silence this warning
sw = Queen.from_dataframe(gdf, silence_warnings=silence_warnings)
queen: 0.2963 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
geos: 0.3076 s; 4 colors
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
/Users/martin/Git/libpysal/libpysal/weights/util.py:1658: FutureWarning: The `query_bulk()` method is deprecated and will be removed in GeoPandas 1.0. You can use the `query()` method instead.
inp, res = gdf.sindex.query_bulk(gdf.geometry, predicate=predicate)
dist1: 0.8972 s; 10 colors
[25]:
times
[25]:
10 | 20 | 30 | 40 | 50 | 60 | 70 | 80 | 90 | 100 | |
---|---|---|---|---|---|---|---|---|---|---|
rook | 0.0031 | 0.0107 | 0.0239 | 0.0462 | 0.0698 | 0.1067 | 0.1493 | 0.1976 | 0.2700 | 0.3191 |
queen | 0.0028 | 0.0099 | 0.0224 | 0.0471 | 0.0683 | 0.1031 | 0.1396 | 0.1864 | 0.2479 | 0.2963 |
geos | 0.0026 | 0.0061 | 0.0131 | 0.0261 | 0.0480 | 0.0729 | 0.1082 | 0.1639 | 0.2187 | 0.3076 |
dist1 | 0.0057 | 0.0188 | 0.0690 | 0.0846 | 0.1344 | 0.2205 | 0.3423 | 0.4713 | 0.6460 | 0.8972 |
[26]:
import matplotlib.pyplot as plt
[27]:
ax = times.T.plot()
ax.set_ylabel("time (s)")
ax.set_xlabel("# of polygons")
locs, labels = plt.xticks()
plt.xticks(ticks=locs, labels=(times.columns ** 2)[:7], rotation="vertical");
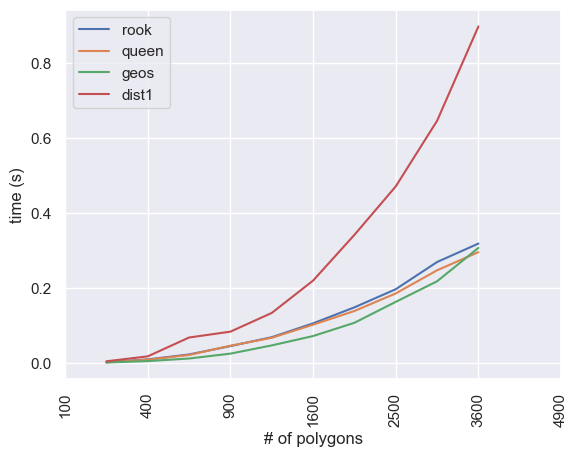
Plotting without the GEOS methods, the difference between queen
and rook
is minimal:
[28]:
ax = times.loc[["rook", "queen"]].T.plot()
ax.set_ylabel("time (s)")
ax.set_xlabel("# of polygons")
locs, labels = plt.xticks()
plt.xticks(ticks=locs, labels=(times.columns ** 2)[:7], rotation="vertical");
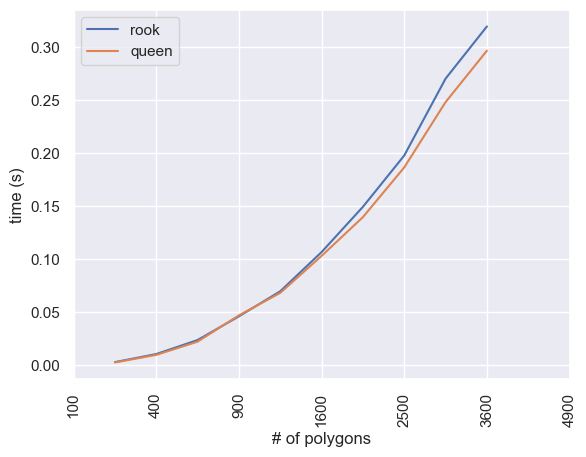
Comparison of strategies¶
Different coloring strategies lead to different results, but also have different performance. It all depends on the prefered goal.
If one want visually balanced result, 'balanced'
strategy could be the right choice. It comes with four different modes of balancing - 'count'
, 'area'
, 'distance'
, and 'centroid'
. The first one attempts to balance the number of features per each color, the second balances the area covered by each color, and the two last are based on the distance between features (either represented by the geometry itself or its centroid, which is a bit faster).
Other strategies might be helpful if one wants to minimize the number of colors as not all strategies use the same amount in the end. Or they just might look better on your map.
[29]:
strategies = [
"balanced",
"largest_first",
"random_sequential",
"smallest_last",
"independent_set",
"connected_sequential_bfs",
"connected_sequential_dfs",
"saturation_largest_first",
]
balanced_modes = ["count", "area", "centroid", "distance"]
[30]:
import libpysal
import warnings
[31]:
sw = libpysal.weights.Queen.from_dataframe(
world, ids=world.index.to_list(), silence_warnings=True
)
Below is a comparison of performance and the result of each of the strategies supported by greedy
.
When using the 'balanced'
strategy with 'area'
, 'distance'
, or 'centroid'
modes, keep in mind that your data needs to be in a projected CRS to obtain correct results. For the simplicity of this comparison, let’s pretend that dataset below is (even though it is not).
Strategies used in mapclassify.greedy
have two origins - 'balanced'
is ported from QGIS while the rest comes from NetworkX. The nippet below generates each option 20x and returns the mean time elapsed together with the number of colors used.
[32]:
times = {}
with warnings.catch_warnings():
warnings.filterwarnings(
"ignore", message="Geometry is in a geographic CRS.", category=UserWarning
)
for strategy in strategies:
if strategy == "balanced":
for mode in balanced_modes:
stgy_mode = strategy + "_" + mode
print(stgy_mode)
kwargs = dict(strategy=strategy, balance=mode, sw=sw)
mean_time, colors = run_greedy(world, kwargs, runs=20)
printer("time", mean_time, numpy.max(colors))
world[stgy_mode], times[stgy_mode] = colors, mean_time
else:
print(strategy)
kwargs = dict(strategy=strategy, sw=sw)
mean_time, colors = run_greedy(world, kwargs, runs=20)
printer("time", mean_time, numpy.max(colors))
world[strategy], times[strategy] = colors, mean_time
balanced_count
time: 0.0005 s; 5 colors
balanced_area
time: 0.0129 s; 5 colors
balanced_centroid
time: 2.1403 s; 5 colors
balanced_distance
time: 2.0867 s; 5 colors
largest_first
time: 0.0056 s; 5 colors
random_sequential
time: 0.0025 s; 5 colors
smallest_last
time: 0.0047 s; 4 colors
independent_set
time: 0.0652 s; 5 colors
connected_sequential_bfs
time: 0.0043 s; 5 colors
connected_sequential_dfs
time: 0.0045 s; 5 colors
saturation_largest_first
time: 0.0405 s; 4 colors
As you can see, smallest_last
and saturation_largest_first
were able, for this particular dataset, to generate greedy coloring using only 4 colors. If one wants to use a higher number than the minimal, the 'balanced'
strategy allows the setting of min_colors
.
[33]:
times = pandas.Series(times)
ax = times.plot(kind="bar")
ax.set_yscale("log")
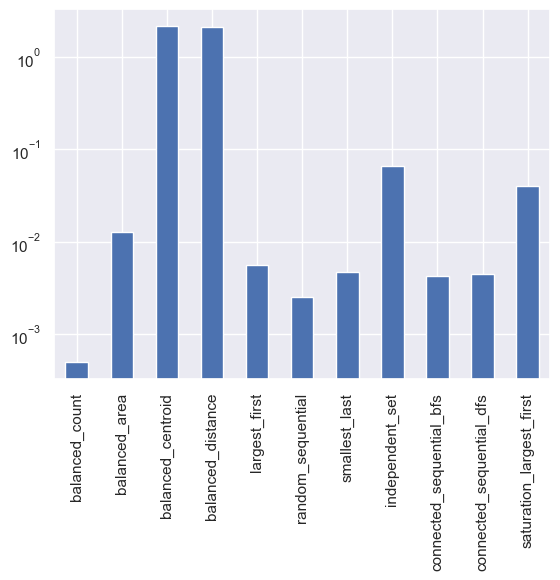
The plot above shows the performance of each strategy. Note that the vertical axis is using log scale.
Below are all results plotted on the map.
[34]:
plt_kws = dict(categorical=True, figsize=(16, 12), cmap="Set3", legend=True)
for strategy in times.index:
ax = world.plot(strategy, **plt_kws)
ax.set_axis_off()
ax.set_title(strategy)
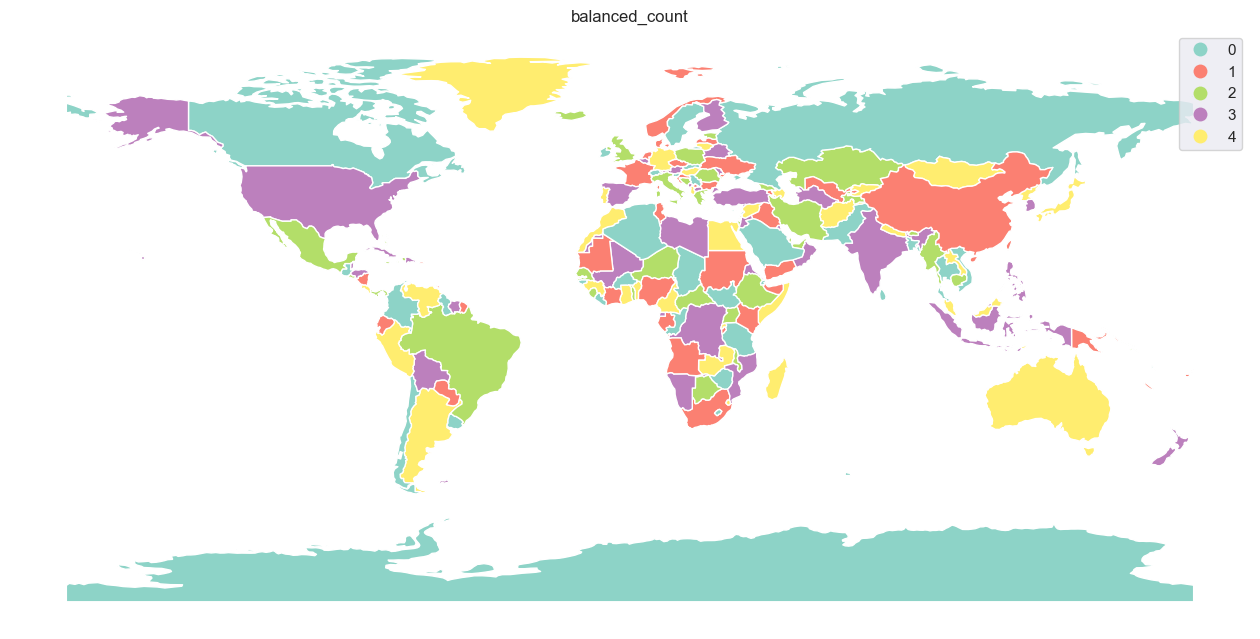
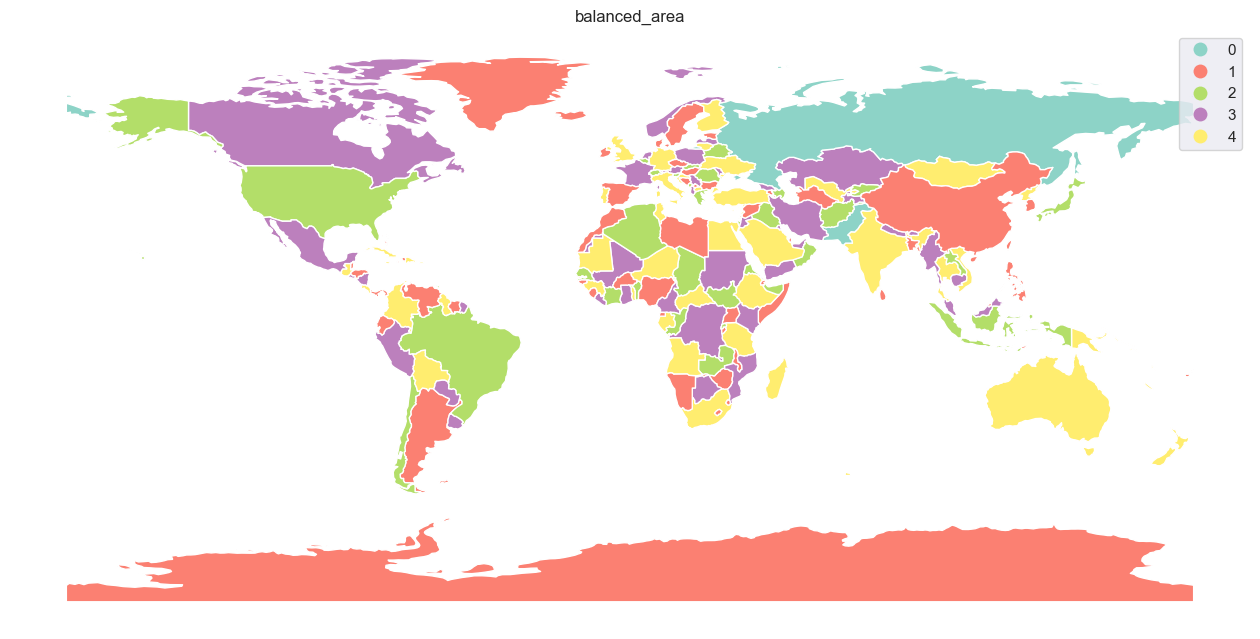
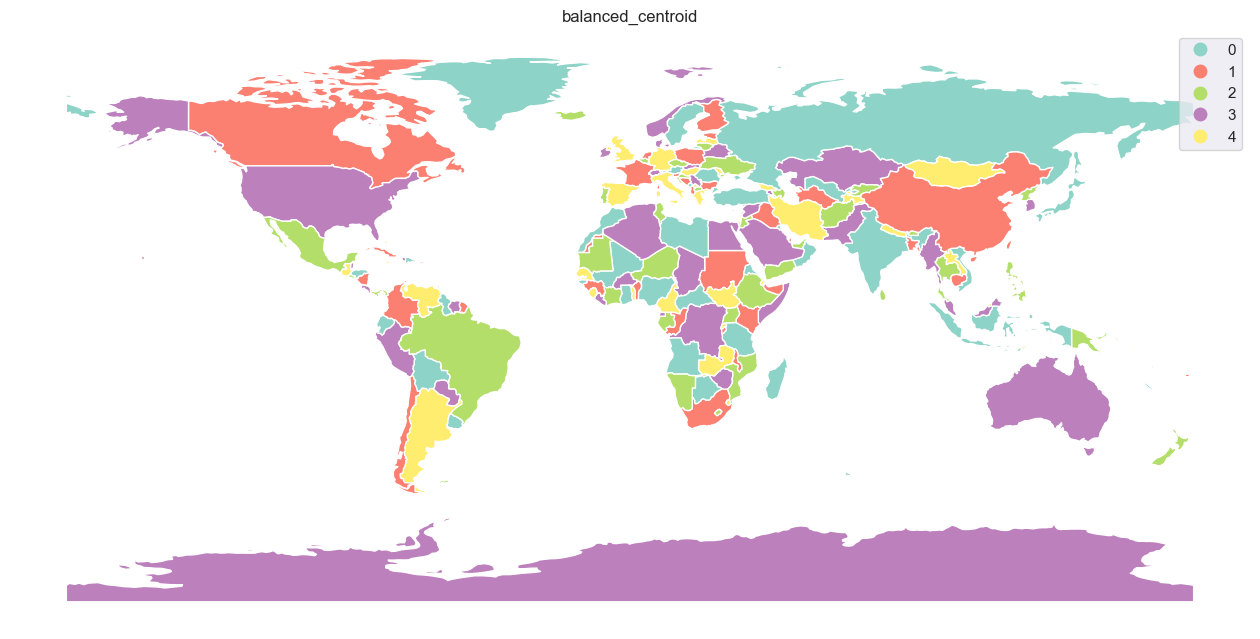
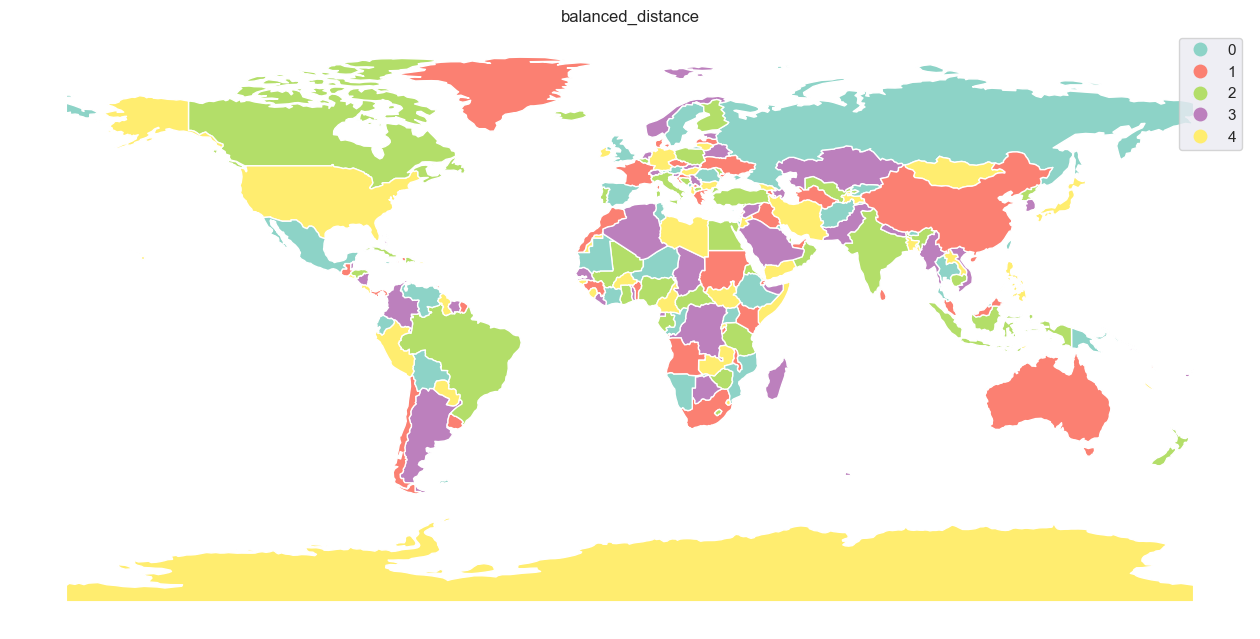
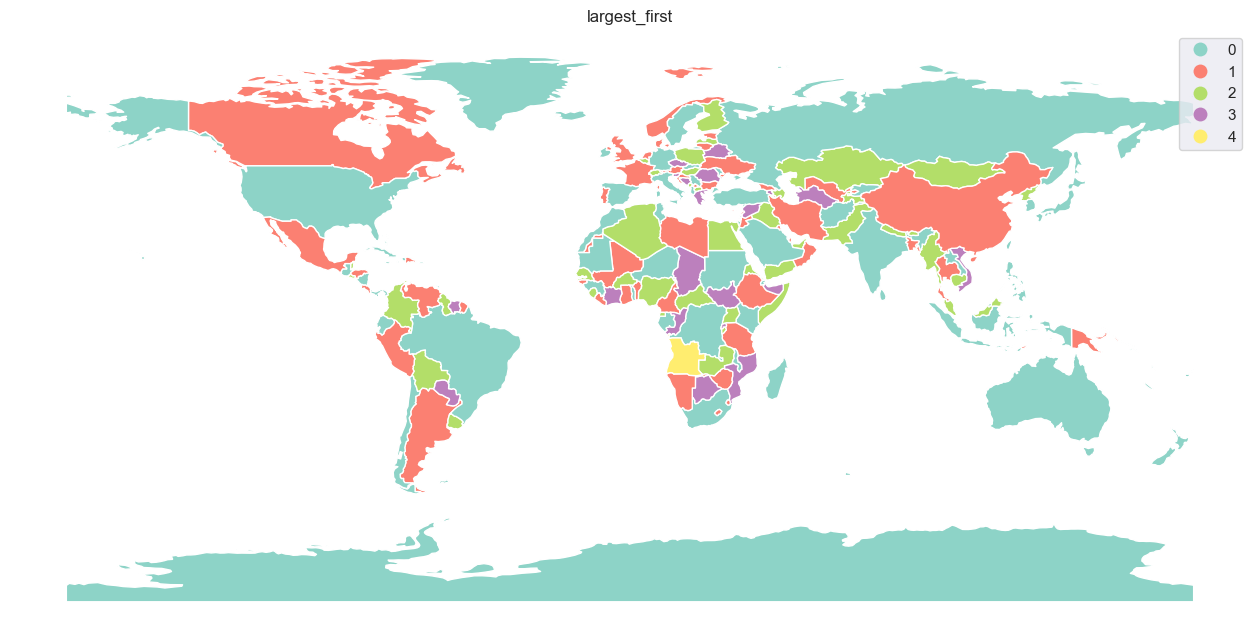
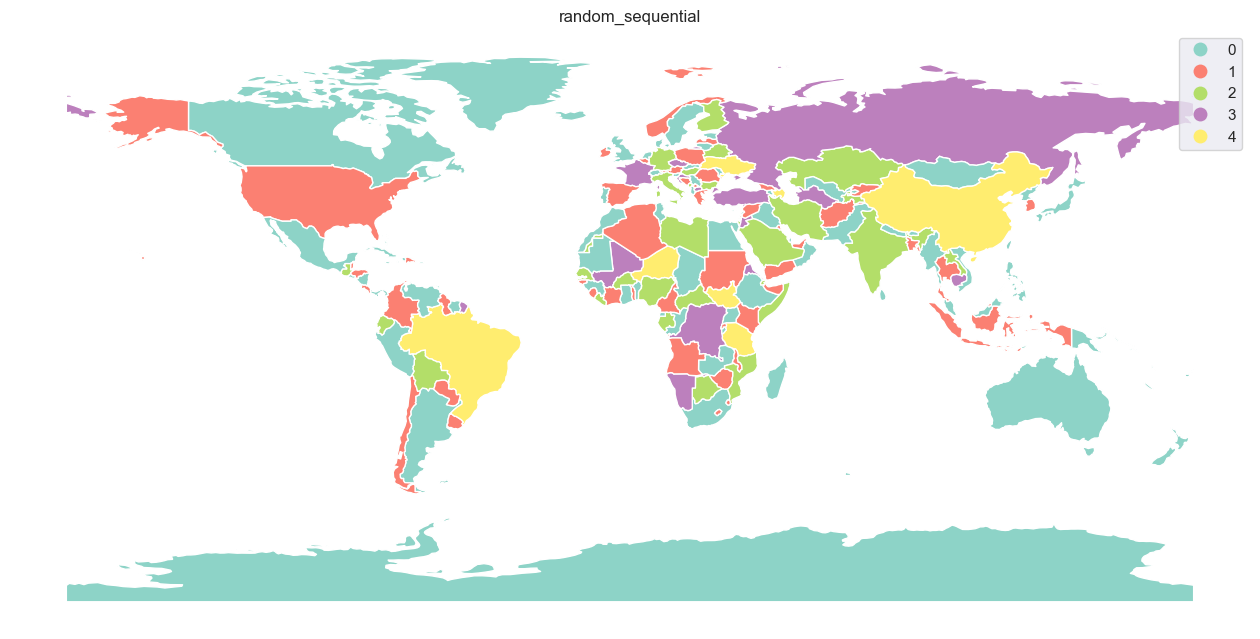
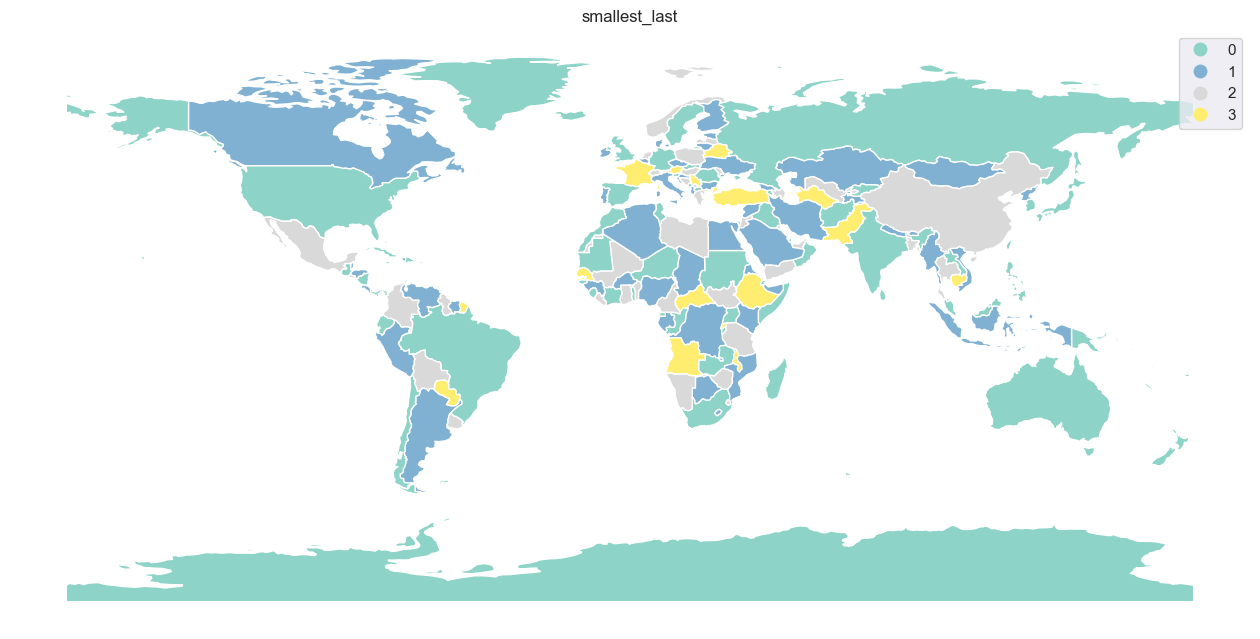
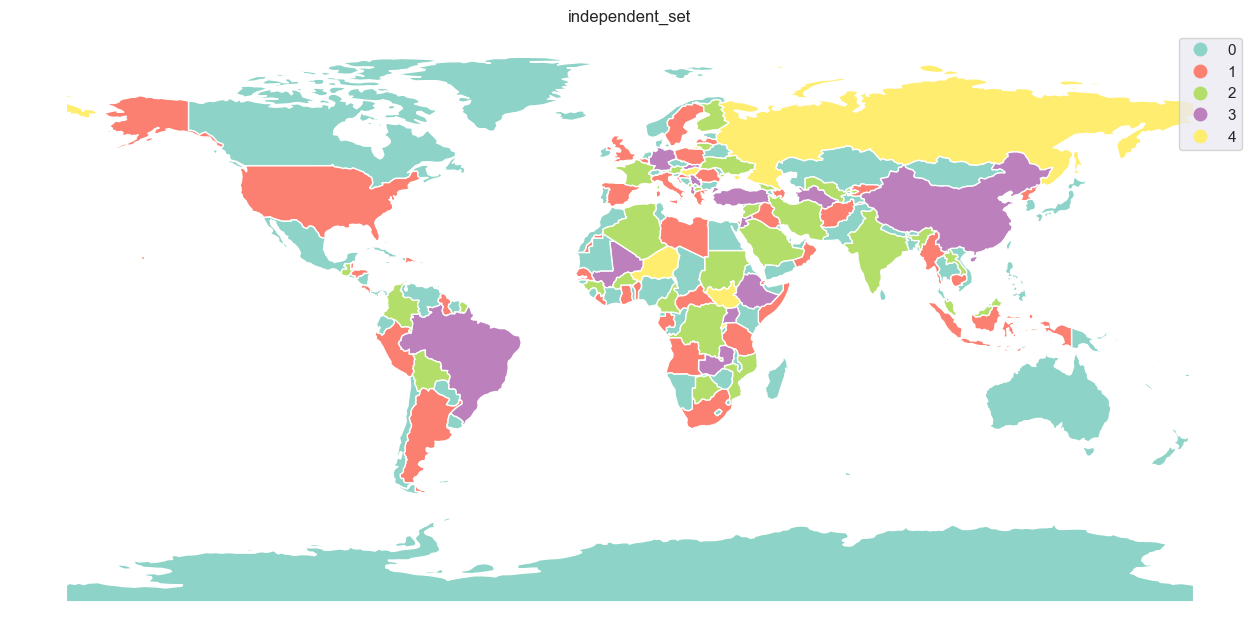
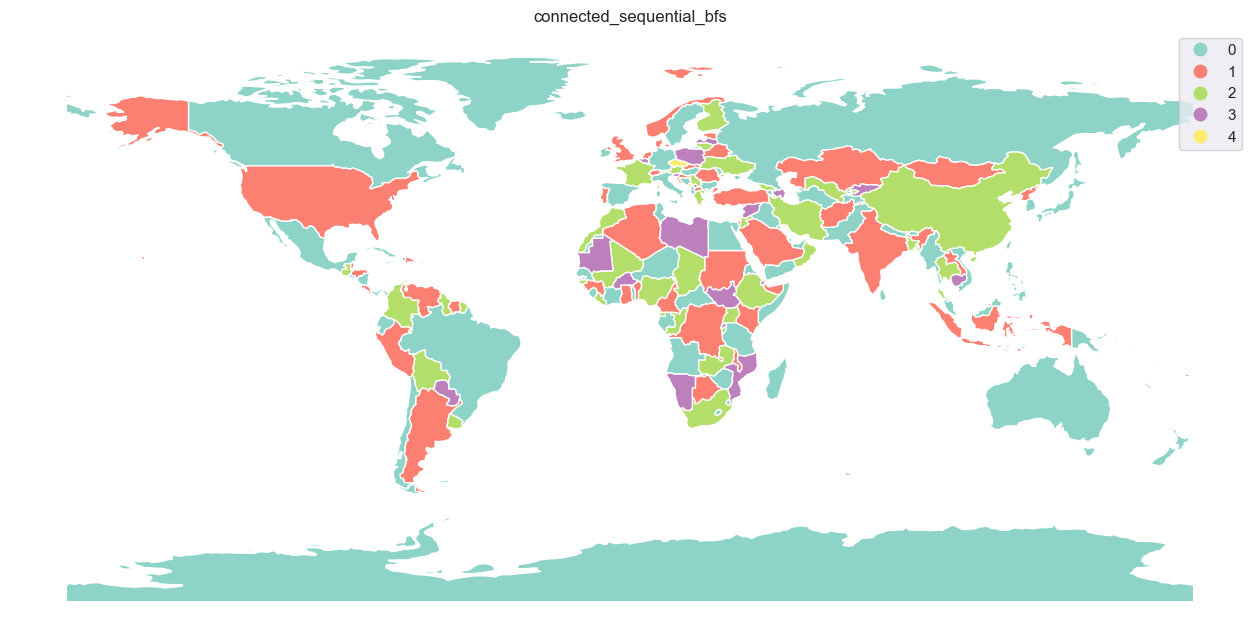
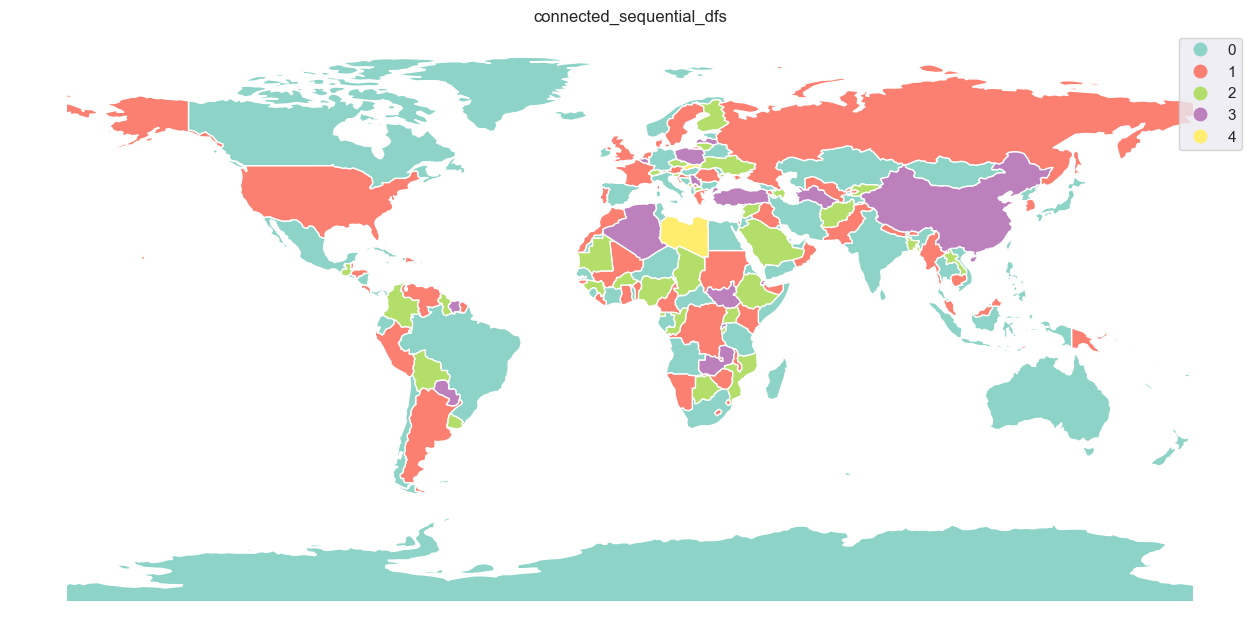
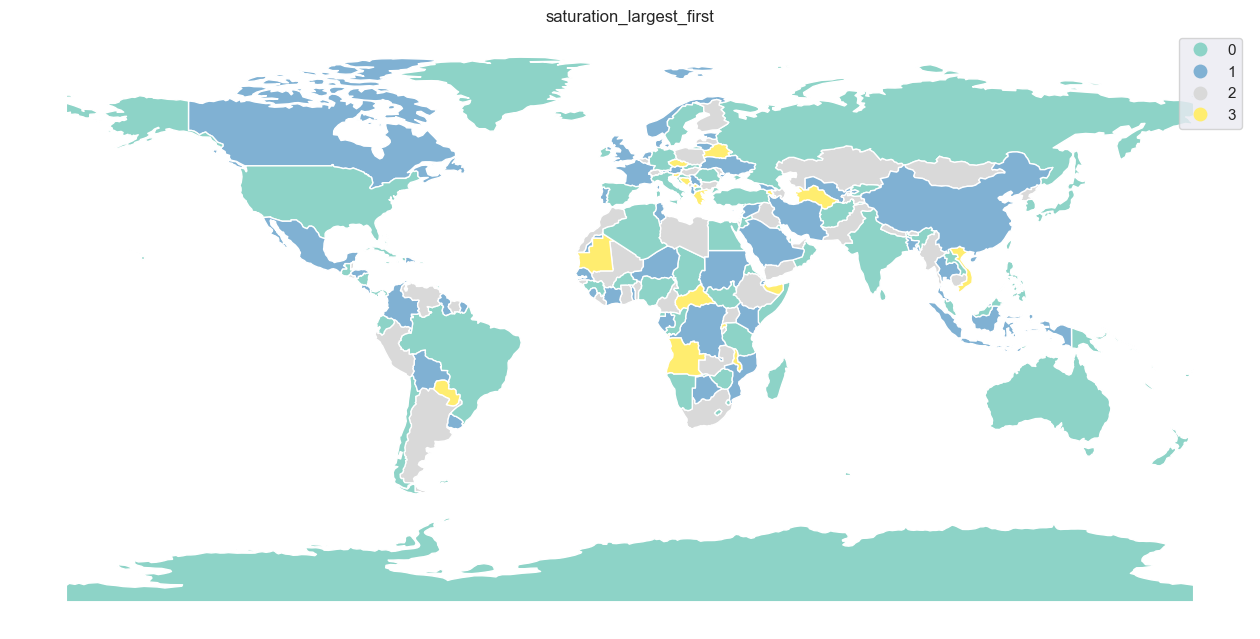